最近ようやくM5Stackも使い慣れてきました。ここいらでちょっとIoT機器として応用させたいと思います。
m5stackからAWSのEC2へデータを飛ばしてブラウザで確認できるようにします。
まずはM5stack側ですが、温湿度、気圧センサーを取り付けます。
https://www.switch-science.com/catalog/6344/
m5stackのスケッチはこちら。設定は下記参照してください。
https://www.conect.plus/post/20210122これでセンサー値を任意のアドレスへMQTT送信できるようになりました。
webサーバー側の開発です。下記を参考に開発しました。
https://hikoleaf.hatenablog.jp/entry/2019/05/18/165038?fbclid=IwAR1S5pqvV9YN1MH-pGN2-WsZpl_aIIiNvcDVfxfzQC0FuRCL0jg1hDA6GnM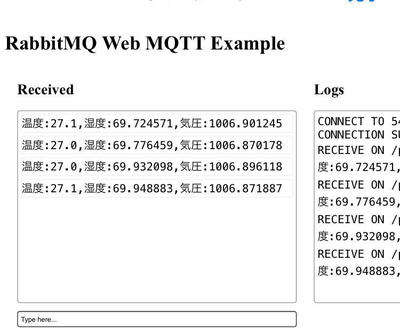
うまくいくとこのようにブラウザでセンサー値が確認できます。
※必ずポートの解放を行ってください。
ざっくりの説明ですが、m5stackとwebサーバーでIoT開発ができました。
慣れればラズパイより使い勝手がいいかもしれません。
今後も精進したいと思います!!